반응형
예외처리 기본
java aaa(.main())
try{
로직(예외발생할만한코드)
}catch(NumberFormatException e){}
ex]exception 예외처리시 오류가 난다면 "import java.lang.Exception;" 시킵니다.
Given : Exception1.java
Given : Exception2.java
참고]익셉션객체는 ArithmeticException이나 NumberFormatException보다
상위 객체이므로 NumberFormatException 대신에 Exception을 넣어줘도 예외처리가 된다.
아래 이미지는 Exception객체의 트리 형식이다.
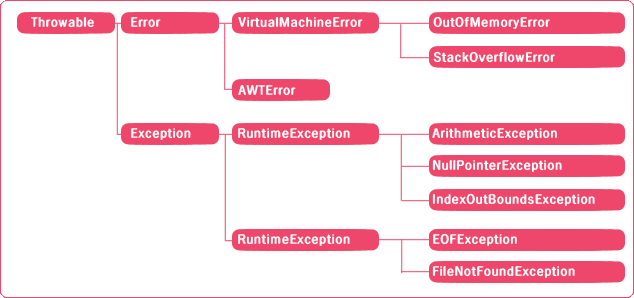
Given : Exception3.java
Given : Exception4.java
Given : Exception5.java
Given : Exception6.java
java aaa(.main())
try{
로직(예외발생할만한코드)
}catch(NumberFormatException e){}
ex]exception 예외처리시 오류가 난다면 "import java.lang.Exception;" 시킵니다.
Given : Exception1.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class Exception1 { public static void main(String[] args){ int a = 0; try{ a = 100; //a에 100을 대입 int arr[] =new int[3]; arr[3] = 4; a = 1000; //a에 1000이 들어가지 않는다. //위 배열에서 지정된 배열이 벗어났으므로 catch로 점프한다. }catch(ArrayIndexOutOfBoundsException e){ System.out.println("배열이 초과되었습니다." + e.getMessage()); } } } |
Given : Exception2.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class Exception2 { public static void main(String[] args){ try{ int a = 0; int num = 1000 / a; System.out.println(num); }catch(Exception e){ System.out.println("0으로 나눌수 없습니다.[∞] : " + e.getMessage()); //.getMessage는 어디서부터 오류가 났는지 알려준다. e.printStackTrace(); //.printStackTrace() 에러가 왜 났는 몇번째 라인에서 났는지 알려준다. } } } |
상위 객체이므로 NumberFormatException 대신에 Exception을 넣어줘도 예외처리가 된다.
아래 이미지는 Exception객체의 트리 형식이다.
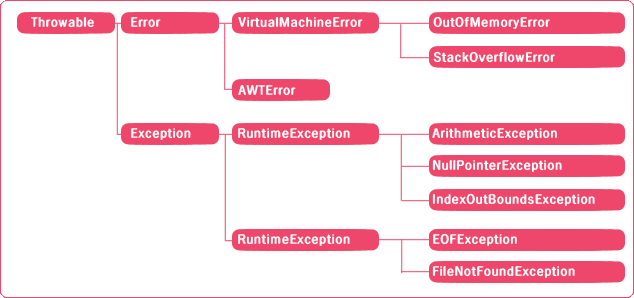
Given : Exception3.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class Exception3 { public static void main(String[] args){ try{ int a = 0; int num = 1000 / a; System.out.println(num); String str="aaa"; int my = Integer.parseInt(str); }catch(ArithmeticException e){ System.out.println("0으로 나눌수 없습니다.[∞] : " + e.getMessage()); }catch(NumberFormatException e){ System.out.println("숫자를 넣어주세요. " + e.getMessage()); }catch(Exception e){ System.out.println("알수 없는 예외" + e.getMessage()); } } } |
Given : Exception4.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class Exception4 { public static void main(String[] args){ try{ int a = 0; int num = 1000 / a; System.out.println(num); String str="aaa"; int my = Integer.parseInt(str); String aaa[] = new String[1]; aaa[0]="안녕"; aaa[1]="없는 방 ㅋ"; //문법적으론 에러는 없지만 방갯수 초과! }catch(Exception e){ System.out.println("알수 없는 예외" + e.getMessage()); } } } |
참고] : 예외가 발생하면 해당 예외 객체가 만들어짐. try()~~catch(){}로 안잡으면
그 메소드를 call한 곳으로 예외 객체가 튕겨나간다.
그 메소드를 call한 곳으로 예외 객체가 튕겨나간다.
Given : Exception5.java
예외처리해주는 두 가지 방식의 예제
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
public class Exception5 { public static void main(String[] args){ Exception5 ex = new Exception5(); ex.myMethod(); } public void myMethod(){ try{ int num = 100/0; System.out.println(num); }catch(Exception e){ System.out.println("알수없는 예외"); } } } ─ 혹은 ──────────────────────────────────── public class Exception5 { public static void main(String[] args){ Exception5 ex = new Exception5(); try{ ex.myMethod(); }catch(Exception e){ System.out.println("알수없는 예외"); } } public void myMethod(){ int num = 100/0; System.out.println(num); } } |
Given : Exception6.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
public class Exception6 { public static void main(String[]args){ String str[] = {"hi","java"}; try{ for(int i=0; i<=str.length;i++){ //바운드익셉션 걸리는 구간 System.out.println(str[i]); } }catch(Exception e){ System.out.println("배열 범위 오류"); //return; 리턴 키워드를 만나도 finally는 실행 //System.exit(0); 시스템 종료를 만났을때는 finally는 실행 안된다. }finally{ //예외가 발생하건 발생하지 않건 무조건 실행 System.out.println("무조건 실행되는 구간"); } System.out.println("zzz"); } } |
참고] : finally 블럭이 try-catch문의 마지막에 추가되면
예외처리가 끝나고도 반드시 finally 블럭이 처리된 다.
이때 중요한 것은 예외가 발생하지 않았을지라도 finally 블럭은 처리된다는 것이다.
또한 try 블럭 안에 return, break, continue 문이 있어도 finally 블럭은 수행된다.
보통 finally 블럭은 finally문 블럭 이전에 열린 소켓과 파일 등을 닫기 위해 사용된다.
예외처리가 끝나고도 반드시 finally 블럭이 처리된 다.
이때 중요한 것은 예외가 발생하지 않았을지라도 finally 블럭은 처리된다는 것이다.
또한 try 블럭 안에 return, break, continue 문이 있어도 finally 블럭은 수행된다.
보통 finally 블럭은 finally문 블럭 이전에 열린 소켓과 파일 등을 닫기 위해 사용된다.
반응형
'Programing > JAVA' 카테고리의 다른 글
자바 :: JAVA :: Thread :: 쓰레드 :: 다중 상속 (1) | 2008.10.20 |
---|---|
JAVA :: 자바 :: 예외처리 :: Encapsulation (0) | 2008.10.20 |
JAVA :: 자바 :: instanceof :: Type 비교연산자 (3) | 2008.10.16 |
JAVA :: 자바 :: 예외처리 :: exception :: try :: catch :: finally :: 사용자 정의 예외클래스 만들기 (0) | 2008.10.16 |
java 세미나 (0) | 2008.10.15 |
윈도우XP에서 jar파일 만들기 (0) | 2008.10.14 |
자바 윈도우 응용프로그램 만들기 :: java -> exe로 만들기! (1) | 2008.10.14 |
Java,Jsp Keywords (0) | 2008.10.14 |